Immutable Value Objects in PHP and Laravel With the Bags Package
Bag is a PHP and Laravel package for Immutable Value Objects, inspired by Spatie's laravel-data package. It can help you create immutable objects to encapsulate your data in a type-safe way with data casts and built-in validation. The package suggests it can be used to replace regular arrays in your code to benefit from type safety. use Bag\Bag; readonly class MyValue extends Bag { public function __construct(public string $name, public int $age) {} } $value = MyValue::from([ 'name' => 'Davey Shafik', 'age' => 40, ]); // Create a new instance with a different value $newValue = $value->with(age: 41); Bag also has an integration with Laravel, using standard Collection and Validation in value objects, as well as Eloquent casts and the ability to inject Bag objects into controllers with validation: use Bag\Attributes\Laravel\FromRouteParameter; use Bag\Bag; class MyValue extends Bag { #[FromRouteParameter()] public string $id; } // MyValue $value will have the populated `id` route parameter class MyController extends Controller { public function store(MyValue $value) { // $value is a validated MyValue object } } This package also has an Artisan command to generate a Bag' using the make:bag` command. Main Features Immutable & Strongly typed Value casting — both input and output Collection support Composable — nest Bag value objects and collections Built-in validation You can get started with Bag by reading the documentation and the source code is available on GitHub at dshafik/bag. The post Immutable Value Objects in PHP and Laravel With the Bags Package appeared first on Laravel News. Join the Laravel Newsletter to get Laravel articles like this directly in your inbox.
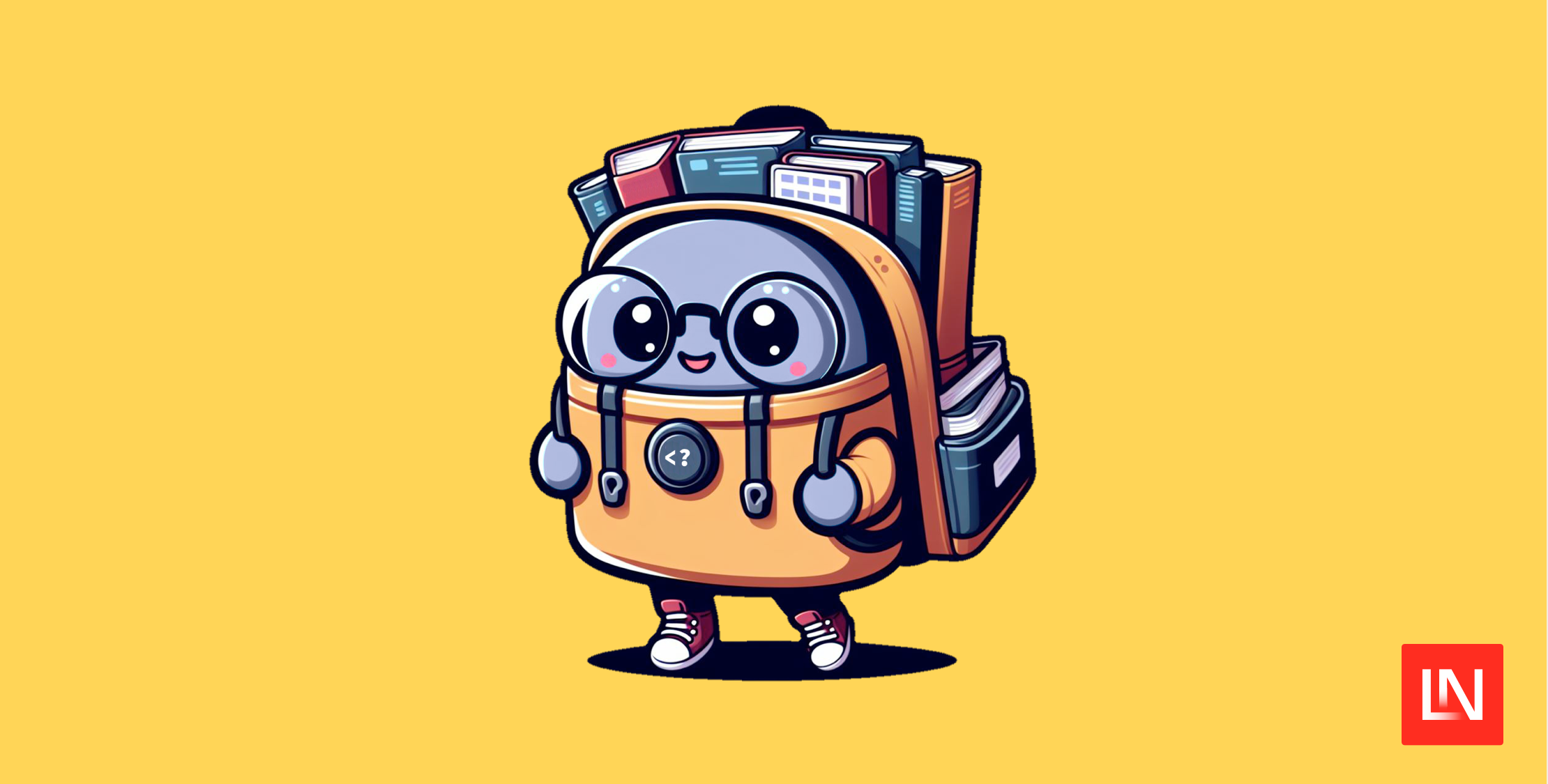
Bag is a PHP and Laravel package for Immutable Value Objects, inspired by Spatie's laravel-data package. It can help you create immutable objects to encapsulate your data in a type-safe way with data casts and built-in validation. The package suggests it can be used to replace regular arrays in your code to benefit from type safety.
use Bag\Bag;
readonly class MyValue extends Bag {
public function __construct(public string $name, public int $age) {}
}
$value = MyValue::from([
'name' => 'Davey Shafik',
'age' => 40,
]);
// Create a new instance with a different value
$newValue = $value->with(age: 41);
Bag also has an integration with Laravel, using standard Collection
and Validation
in value objects, as well as Eloquent casts and the ability to inject Bag
objects into controllers with validation:
use Bag\Attributes\Laravel\FromRouteParameter;
use Bag\Bag;
class MyValue extends Bag
{
#[FromRouteParameter()]
public string $id;
}
// MyValue $value will have the populated `id` route parameter
class MyController extends Controller {
public function store(MyValue $value) {
// $value is a validated MyValue object
}
}
This package also has an Artisan command to generate a Bag' using the
make:bag` command.
Main Features
- Immutable & Strongly typed
- Value casting — both input and output
- Collection support
- Composable — nest Bag value objects and collections
- Built-in validation
You can get started with Bag by reading the documentation and the source code is available on GitHub at dshafik/bag.
The post Immutable Value Objects in PHP and Laravel With the Bags Package appeared first on Laravel News.
Join the Laravel Newsletter to get all the latest Laravel articles like this directly in your inbox.
What's Your Reaction?
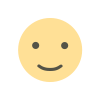
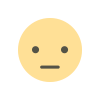
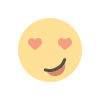
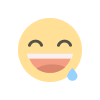
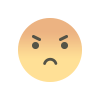
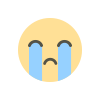
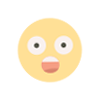